Fibonacci series in C programming: C program for Fibonacci series using a loop and recursion. Using the code below you can print as many terms of the series as required. Numbers of this sequence are known as Fibonacci numbers. The first few numbers of the series are 0, 1, 1, 2, 3, 5, 8, ...,. Except for the first two terms of the sequence, every other term is the sum of the previous two terms, for example, 8 = 3 + 5 (addition of 3 and 5).
Fibonacci series C program using a for loop
/* Fibonacci series program in C language */
#include
int main()
{
int n, first = 0, second = 1, next, c;
printf("Enter the number of terms\n");
scanf("%d", &n);
printf("First %d terms of Fibonacci series are:\n", n);
for (c = 0; c < n; c++)
{
if (c <= 1)
next = c;
else
{
next = first + second;
first = second;
second = next;
}
printf("%d\n", next);
}
return 0;
}
#include
int main()
{
int n, first = 0, second = 1, next, c;
printf("Enter the number of terms\n");
scanf("%d", &n);
printf("First %d terms of Fibonacci series are:\n", n);
for (c = 0; c < n; c++)
{
if (c <= 1)
next = c;
else
{
next = first + second;
first = second;
second = next;
}
printf("%d\n", next);
}
return 0;
}
Output of program:
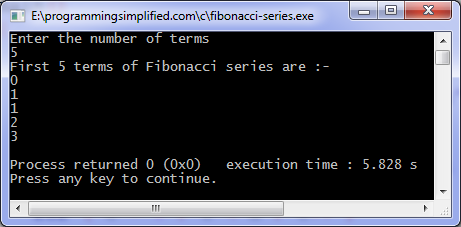
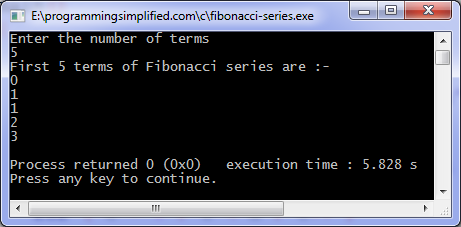
Fibonacci series C program using recursion
#include
int f(int);
int main()
{
int n, i = 0, c;
scanf("%d", &n);
printf("Fibonacci series terms are:\n");
for (c = 1; c <= n; c++)
{
printf("%d\n", f(i));
i++;
}
return 0;
}
int f(int n)
{
if (n == 0 || n == 1)
return n;
else
return (f(n-1) + f(n-2));
}
int f(int);
int main()
{
int n, i = 0, c;
scanf("%d", &n);
printf("Fibonacci series terms are:\n");
for (c = 1; c <= n; c++)
{
printf("%d\n", f(i));
i++;
}
return 0;
}
int f(int n)
{
if (n == 0 || n == 1)
return n;
else
return (f(n-1) + f(n-2));
}
No comments:
Post a Comment